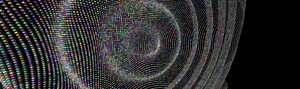
A very brief introduction to luma.gl
What is luma.gl?
It is a framework for GPU-based Visualization and Computation powered by WebGl2.
It is a JavaScript framework that provides developers easier and complete access to underlying WebGL2 APIs provided by modern browsers.
luma.gl was originally created in late 2015 as a fork of PhiloGL to provide high performance WebGL rendering capability for deck.gl - a 3D visualization framework for large scale data.
The core philosophy of luma.gl is to give developers full access to WebGL2 with a strong focus on performance. And the core use case for luma.gl is visualization of large datasets.
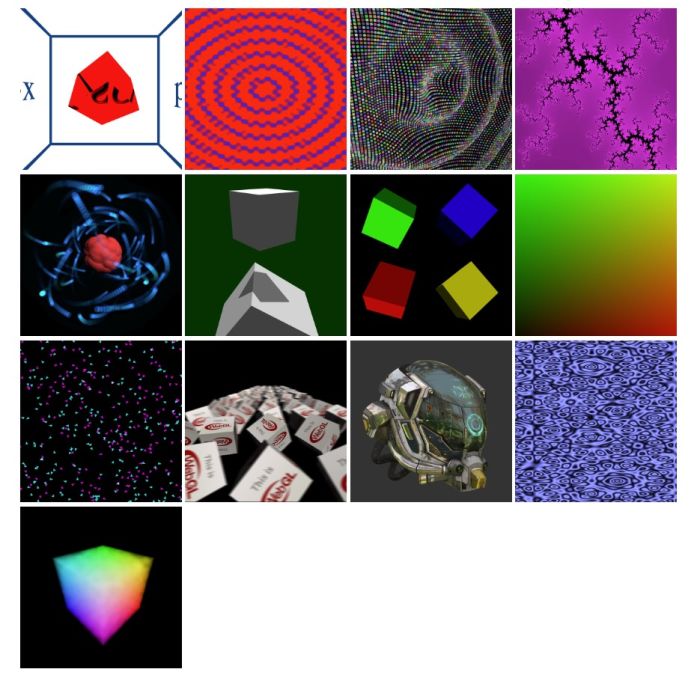
Link to core examples page: https://luma.gl/#/examples/overview
Getting Started
Install luma.gl using yarn
npm
can also be used as a drop-in substitute in most cases.
Using with Node.js
luma.gl runs using headless-gl under Node.js which is useful for unit testing. We need to use yarn install gl
to install headless-gl
which will be automatically luma.gl under Node.js.
We can now create a context using the createGLContext
context function.
Shader Tools
To add/inject existing modules into your shaders, just add the modules parameter to your assembleShaders
call:
To create a new shader module, you need to create a descriptor object.
This object can be used as shader module directly:
Comments